第13章 SupportFunctions的使用(一) 本期教程主要讲解支持函数中的数据拷贝,数据赋值和浮点数转换为定点数。
13.1 数据拷贝Copy
13.2 数据填充Fill
13.3 浮点数转定点数 Float to Fix
13.4 总结
13.1 数据拷贝Copy
这部分函数用于数据拷贝,公式描述如下:
pDst[n] =pSrc[n]; 0 <= n < blockSize.
13.1.1 arm_copy_f32函数定义如下:
voidarm_copy_f32(float32_t * pSrc, float32_t * pDst, uint32_t blockSize)
参数定义:
[in] *pSrc points to inputvector
[out] *pDst points to outputvector
[in] blockSizelength of the input vector
13.1.2 arm_copy_q31此函数的使用比较简单,函数定义如下:
void arm_copy_q31(q31_t * pSrc, q31_t * pDst, uint32_tblockSize)
参数定义:
[in] *pSrc points to inputvector
[out] *pDst points to outputvector
[in] blockSizelength of the input vector
13.1.3 arm_copy_q15函数定义如下:
void arm_copy_q15(q15_t * pSrc, q15_t * pDst, uint32_tblockSize)
参数定义:
[in] *pSrc points to inputvector
[out] *pDst points to outputvector
[in] blockSizelength of the input vector
13.1.4 arm_copy_q7函数定义如下:
void arm_copy_q7(q7_t * pSrc, 7_t * pDst, int32_tblockSize)
参数定义:
[in] *pSrc points to inputvector
[out] *pDst points to outputvector
[in] blockSizelength of the input vector
13.1.5 实例讲解实验目的:
1. 学习SupportFunctions中的数据拷贝
实验内容:
1. 按下按键K1, 串口打印函数DSP_Copy的输出结果
实验现象:
通过窗口上位机软件SecureCRT(V5光盘里面有此软件)查看打印信息现象如下:

程序设计:
- /*
- *********************************************************************************************************
- * 函 数 名: DSP_Copy
- * 功能说明: 数据拷贝
- * 形 参:无
- * 返 回 值: 无
- *********************************************************************************************************
- */
- static void DSP_Copy(void)
- {
- float32_t pSrc[10] = {0.6557, 0.0357, 0.8491, 0.9340, 0.6787, 0.7577, 0.7431, 0.3922, 0.6555, 0.1712};
- float32_t pDst[10];
- uint32_t pIndex;
-
- q31_t pSrc1[10];
- q31_t pDst1[10];
-
- q15_t pSrc2[10];
- q15_t pDst2[10];
-
- q7_t pSrc3[10];
- q7_t pDst3[10];
-
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("pSrc[%d] = %f\r\n", pIndex, pSrc[pIndex]);
- }
- arm_copy_f32(pSrc, pDst, 10);
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("arm_copy_f32: pDst[%d] = %f\r\n", pIndex, pDst[pIndex]);
- }
-
- /*****************************************************************/
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- pSrc1[pIndex] = rand();
- printf("pSrc1[%d] = %d\r\n", pIndex, pSrc1[pIndex]);
- }
- arm_copy_q31(pSrc1, pDst1, 10);
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("arm_copy_q31: pDst1[%d] = %d\r\n", pIndex, pDst1[pIndex]);
- }
- /*****************************************************************/
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- pSrc2[pIndex] = rand()%32768;
- printf("pSrc2[%d] = %d\r\n", pIndex, pSrc2[pIndex]);
- }
- arm_copy_q15(pSrc2, pDst2, 10);
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("arm_copy_q15: pDst2[%d] = %d\r\n", pIndex, pDst2[pIndex]);
- }
- /*****************************************************************/
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- pSrc3[pIndex] = rand()%128;
- printf("pSrc3[%d] = %d\r\n", pIndex, pSrc3[pIndex]);
- }
- arm_copy_q7(pSrc3, pDst3, 10);
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("arm_copy_q7: pDst3[%d] = %d\r\n", pIndex, pDst3[pIndex]);
- }
- /*****************************************************************/
- printf("******************************************************************\r\n");
- }
复制代码
13.2 数据填充Fill
这部分函数用于数据填充,公式描述如下:
pDst[n] =value; 0 <= n < blockSize.
13.2.1 arm_fill_f32函数定义如下:
void arm_fill_f32(float32_t value, float32_t * pDst, uint32_tblockSize)
参数定义:
[in] value input value to be filled
[out] *pDst points to output vector
[in] blockSize length of the outputvector
13.2.2 arm_fill_q31此函数的使用比较简单,函数定义如下:
void arm_fill_q31(q31_t value, q31_t * pDst, uint32_tblockSize)
参数定义:
[in] value input value to be filled
[out] *pDst points to output vector
[in] blockSize length of the outputvector
13.2.3 arm_fill_q15函数定义如下:
void arm_fill_q15(q15_t value, q15_t * pDst, uint32_tblockSize)
参数定义:
[in] value input value to be filled
[out] *pDst points to output vector
[in] blockSize length of the outputvector
13.2.4 arm_fill_q7函数定义如下:
void arm_fill_q7(q7_t value, q7_t * pDst, uint32_tblockSize)
参数定义:
[in] value input value to be filled
[out] *pDst points to output vector
[in] blockSize length of the outputvector
13.2.5 实例讲解实验目的:
1. 学习SupportFunctions中的数据填充
实验内容:
1. 按下按键K2, 串口打印函数DSP_Fill的输出结果
实验现象:
通过窗口上位机软件SecureCRT(V5光盘里面有此软件)查看打印信息现象如下:

程序设计:
- /*
- *********************************************************************************************************
- * 函 数 名: DSP_Fill
- * 功能说明: 数据填充
- * 形 参:无
- * 返 回 值: 无
- *********************************************************************************************************
- */
- static void DSP_Fill(void)
- {
- float32_t pDst[10];
- uint32_t pIndex;
-
- q31_t pDst1[10];
-
- q15_t pDst2[10];
-
- q7_t pDst3[10];
-
-
- arm_fill_f32(3.33f, pDst, 10);
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("arm_fill_f32: pDst[%d] = %f\r\n", pIndex, pDst[pIndex]);
- }
-
- /*****************************************************************/
- arm_fill_q31(0x11111111, pDst1, 10);
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("arm_fill_q31: pDst1[%d] = %x\r\n", pIndex, pDst1[pIndex]);
- }
- /*****************************************************************/
- arm_fill_q15(0x1111, pDst2, 10);
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("arm_fill_q15: pDst2[%d] = %x\r\n", pIndex, pDst2[pIndex]);
- }
- /*****************************************************************/
- arm_fill_q7(0x11, pDst3, 10);
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("arm_fill_q7: pDst3[%d] = %x\r\n", pIndex, pDst3[pIndex]);
- }
- /*****************************************************************/
- printf("******************************************************************\r\n");
- }
复制代码
13.3 浮点数转定点数Float to Fix
13.3.1 arm_float_to_q31
公式描述:
pDst[n] = (q31_t)(pSrc[n] * 2147483648); 0 <= n < blockSize
函数定义如下:
void arm_float_to_q31(float32_t * pSrc, q31_t * pDst, uint32_tblockSize)
参数定义:
[in] *pSrc points to the floating-point inputvector
[out] *pDst points to the Q31 output vector
[in] blockSize length of the input vector
注意事项:
1. 这个函数使用了饱和运算。
2. 输出结果的范围是[0x80000000 0x7FFFFFFF]
13.3.2 arm_float_to_q15公式描述:
pDst[n] = (q15_t)(pSrc[n] * 32768); 0 <= n < blockSize.
函数定义如下:
void arm_float_to_q15(float32_t * pSrc, q15_t * pDst, uint32_tblockSize)
参数定义:
[in] *pSrc points to the floating-point inputvector
[out] *pDst points to the Q15 output vector
[in] blockSize length of the input vector
注意事项:
1. 这个函数使用了饱和运算。
2. 输出结果的范围是[0x8000 0x7FFF]
13.3.3 arm_float_to_q7公式描述:
pDst[n] = (q7_t)(pSrc[n] * 128); 0 <= n < blockSize.
函数定义如下:
void arm_float_to_q7(float32_t * pSrc, q7_t * pDst, uint32_tblockSize)
参数定义:
[in] *pSrc points to the floating-point inputvector
[out] *pDst points to the Q7 output vector
[in] blockSize length of the input vector
注意事项:
1. 这个函数使用了饱和运算。
2. 输出结果的范围是[0x80 0x7F]
13.3.4 实例讲解实验目的:
1. 学习SupportFunctions中的浮点数转定点数
实验内容:
1. 按下按键K3, 串口打印函数DSP_FloatToFix的输出结果
实验现象:
通过窗口上位机软件SecureCRT(V5光盘里面有此软件)查看打印信息现象如下:
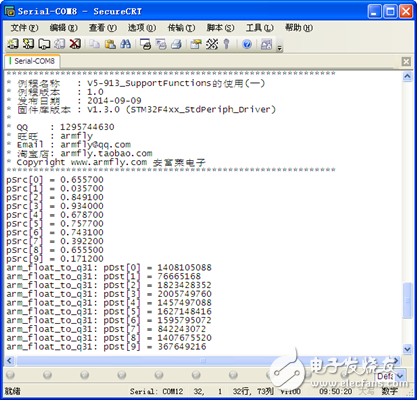
程序设计:
- /*
- *********************************************************************************************************
- * 函 数 名: DSP_FloatToFix
- * 功能说明: 浮点数转定点数
- * 形 参:无
- * 返 回 值: 无
- *********************************************************************************************************
- */
- static void DSP_FloatToFix(void)
- {
- float32_t pSrc[10] = {0.6557, 0.0357, 0.8491, 0.9340, 0.6787, 0.7577, 0.7431, 0.3922, 0.6555, 0.1712};
- uint32_t pIndex;
-
- q31_t pDst1[10];
- q15_t pDst2[10];
- q7_t pDst3[10];
-
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("pSrc[%d] = %f\r\n", pIndex, pSrc[pIndex]);
- }
-
- /*****************************************************************/
- arm_float_to_q31(pSrc, pDst1, 10);
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("arm_float_to_q31: pDst[%d] = %d\r\n", pIndex, pDst1[pIndex]);
- }
-
- /*****************************************************************/
- arm_float_to_q15(pSrc, pDst2, 10);
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("arm_float_to_q15: pDst1[%d] = %d\r\n", pIndex, pDst2[pIndex]);
- }
-
- /*****************************************************************/
- arm_float_to_q7(pSrc, pDst3, 10);
- for(pIndex = 0; pIndex < 10; pIndex++)
- {
- printf("arm_float_to_q7: pDst2[%d] = %d\r\n", pIndex, pDst3[pIndex]);
- }
- /*****************************************************************/
- printf("******************************************************************\r\n");
- }
复制代码
13.4 总结
本期教程就跟大家讲这么多,有兴趣的可以深入研究这些函数源码的实现。